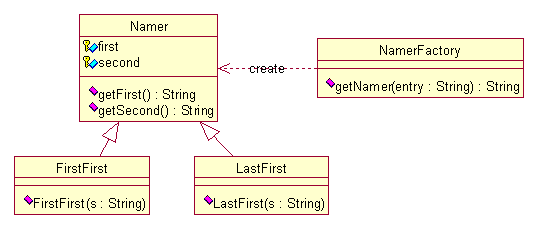
ex) 입력란에 성, 이름을 받아 그것을 유효한 성과 이름으로 나누어 저장한다.
------------------------------------------------------------------------------------
- super class : 입력값에 대한 어떠한 연산 없이 변수 저장, child class에서 멤버변수를 사용하므로 protected로 설정한다.
public class Namer {
//base class extended by two child classes
protected String last; //split name
protected String first; //stored here
public String getFirst() {
return first; //return first nmae
}
public String getLast() {
return last; //return last name
}
}
------------------------------------------------------------------------------------
- child class1 : 인덱스로 짜른 첫 String이 성(First)라고 생각한다 공백으로 구분
public class FirstFirst extends Namer {
//extracts first name from last name
//when separated by a space
public FirstFirst(String s) {
int i = s.lastIndexOf(" "); //find sep space
if (i>0) {
first = s.substring(0, i).trim();
last =s.substring(i+1).trim();
} else {
first = ""; // if no space
last = s; // put all in last name
}
}
}
- child class2 : 인덱스로 짜른 뒤 String이 성(First)라고 생각한다 , 으로 구분(미국사람들이 이렇게 쓰나 보다. 김 개똥 또는 개똥,김 이란 말이겠지.)
public class LastFirst extends Namer {
// extracts last name from first name
// when separated by a comma
public LastFirst(String s) {
int i = s.indexOf(","); //find comma
if (i > 0) {
last = s.substring(0, i).trim();
first = s.substring(i + 1).trim();
} else {
last = s; //if no comma,
first = ""; //put all in last name
}
}
}
------------------------------------------------------------------------------------
- Factory class
public class NamerFactory {
//Factory decides which class to return based on
//presence of a comma
public Namer getNamer(String entry) {
//comma determines name order
int i = entry.indexOf(",");
if (i > 0)
return new LastFirst(entry);
else
return new FirstFirst(entry);
}
}
------------------------------------------------------------------------------------
return 받은 Namer 값은 first와 last 변수에 유효한 값이 저장되어 있으므로 어떤 클래스를 리턴하는지는 상관없이 클래스 인스턴스의 메소드를 호출할 수 있다.
[출처] [패턴] 생성 패턴 : Simple Factory Pattern|작성자 그린
'Design Patterns' 카테고리의 다른 글
[생성 패턴] 싱글톤(Singleton) 패턴 (0) | 2009.02.04 |
---|